Keywords : ultrasonic sensor, Arduino, HC-SR04, ultrasonic library, tinkercad
What is ultrasonic distance measuring
Measuring distance using ultrasonic technology is just a one application of ultrasonic technology. (There are other applications like ultrasound scanning, ultrasonic cleaning, ultrasonic polishing etc,)
Ultrasonic sensors are often called as Sonar sensors and we can use them for many applications like,
- Distance measuring (Robotics applications,Parking applications, Collision detection)
- Depth and level monitoring (Liquid level monitoring)
- Object presence detection (Home automation, Automated parking, Security Applications, Light Management Systems etc)
In this article we will see how to use cheap HC-SR04 sensor along with an Arduino to measure distance with an object. It is really important to note that there are various types if ultrasonic sensors which can be used for different applications. Ultrasonic sensors work on the range above the range which human ears can sense.(20 Hz - 20 kHz) The sensor we are going to use ( HC-SR04 ) will work on the range of 40 kHz.
Lets see the working principle of ultrasonic sensor.
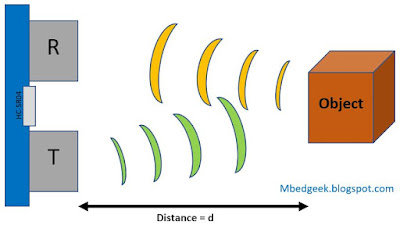 |
How Ultrasonic sensor works |
Transmitter part of the sensor send an ultrasonic signal which will bounce back with the obstacles on its way. Receiver detects the echo of the ultrasonic wave and gives us a feedback. Since we can measure the time gap between the trigger signal and echo signal we can measure the distance to the obstacle. (for normal calculations we take the speed of sound in Air as 340 m/s.
As per the datasheet we can use ultrasonic.h library.
How to download the library.
(And then unzip and copy it into your Arduino Library folder, ex: C:\Program Files (x86)\Arduino\libraries
2) Or else you can download the ultrasonic sensor library as below.
go to sketch >> Include Library >> Manage Libraries
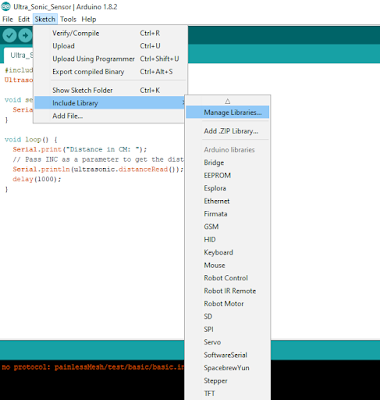 |
Installing ultrasonic Library - 1 |
And then, wait few seconds to update the Arduino libraries and type "ultrasonic.h" and press "enter" in the search bar.
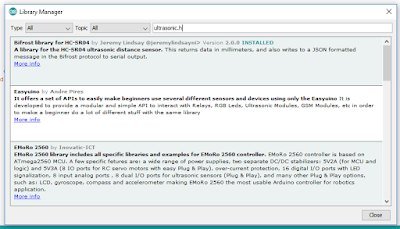 |
Installing ultrasonic Library - 2 |
Go down and find the below library and click Install. "Ultrasonic by Erick Simoes"
 |
Installing ultrasonic Library - 3 |
OK. Now you are good to use ultrasonic library.
Lets connect Arduino Uno with Ultrasonic Sensor
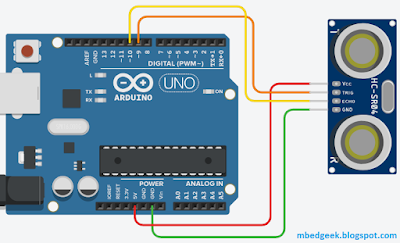 |
Arduino Uno and HC-SR04 connection |
<ARDUINO CODE> //Print distance in cm, using the ultrasonic library
#include <Ultrasonic.h>
const byte trigger_pin = 9;
const byte echo_pin = 10;
Ultrasonic ultrasonic(trigger_pin, echo_pin); //(initialize the library with connections)
void setup() {
Serial.begin(9600);
}
void loop() {
Serial.print("Distance (cm) : ");
Serial.println(ultrasonic.distanceRead()); //print data in cm
delay(100);
}
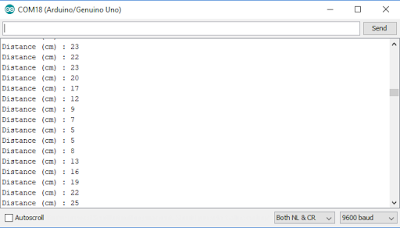 |
Print Ultrasonic Data in Centimeters |
<ARDUINO CODE> //Print distance in inches, using the ultrasonic library
#include <Ultrasonic.h>
const byte trigger_pin = 9;
const byte echo_pin = 10;
Ultrasonic ultrasonic(trigger_pin, echo_pin); //(initialize the library with connections)
void setup() {
Serial.begin(9600);
}
void loop() {
Serial.print("Distance (inches) : ");
Serial.println(ultrasonic.distanceRead(INC)); //print data in inches
delay(100);
}
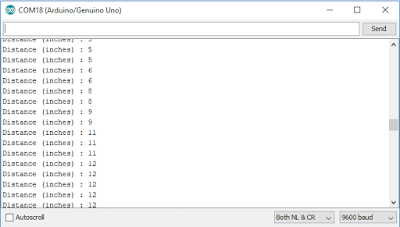 |
Print Ultrasonic Data in Inches |
Ultrasonic sensor can also be easily implemented without ultrasonic.h library. Since ultrasonic library is not available in "tinkercad" I will simulate it using "tinkercad".
<ARDUINO CODE> //Print distance in cm, without using the ultrasonic library
const byte trigger_Pin = 9; //Initialize I/O pins
const byte echo_Pin = 10;
unsigned long duration; //Since PulseIn return an unsigned Long
unsigned int distance; //To save the distance
void setup() {
Serial.begin(9600); //Initialize Serial communication
pinMode(echo_Pin, INPUT); //Echo pin as Input
pinMode(trigger_Pin, OUTPUT); //Trigger pin as Output
}
void loop() {
digitalWrite(trigger_Pin, LOW); //Make Trigger pin Low at start
delay(1);
digitalWrite(trigger_Pin, HIGH);
delayMicroseconds(10); //Make Trigger pin High for 10 uS to start sending the pulse
digitalWrite(trigger_Pin, LOW);
duration = pulseIn(echo_Pin, HIGH); //Save the time it took ultrasonic wave to come back
distance = duration * 0.017; //((340*100)/10e6)/2
/* Speed of the sound in Air = 340 m/S
* multiply it by 100 to get the data in cm
* divide by 1,000,000 as duration is measured in microseconds
* divide by 2 as ultrasound signal travels to object and comes back
*/
Serial.print("Distance (cm) : ");
Serial.println(distance);
delay(100);
}